Metrics Plugin
The Metrics Plugin is used to gather timing information about Player’s execution of a flow. There are also platform specific integrations to include
render
and update
times.The diagram below illistrates some of the timing information gathered:
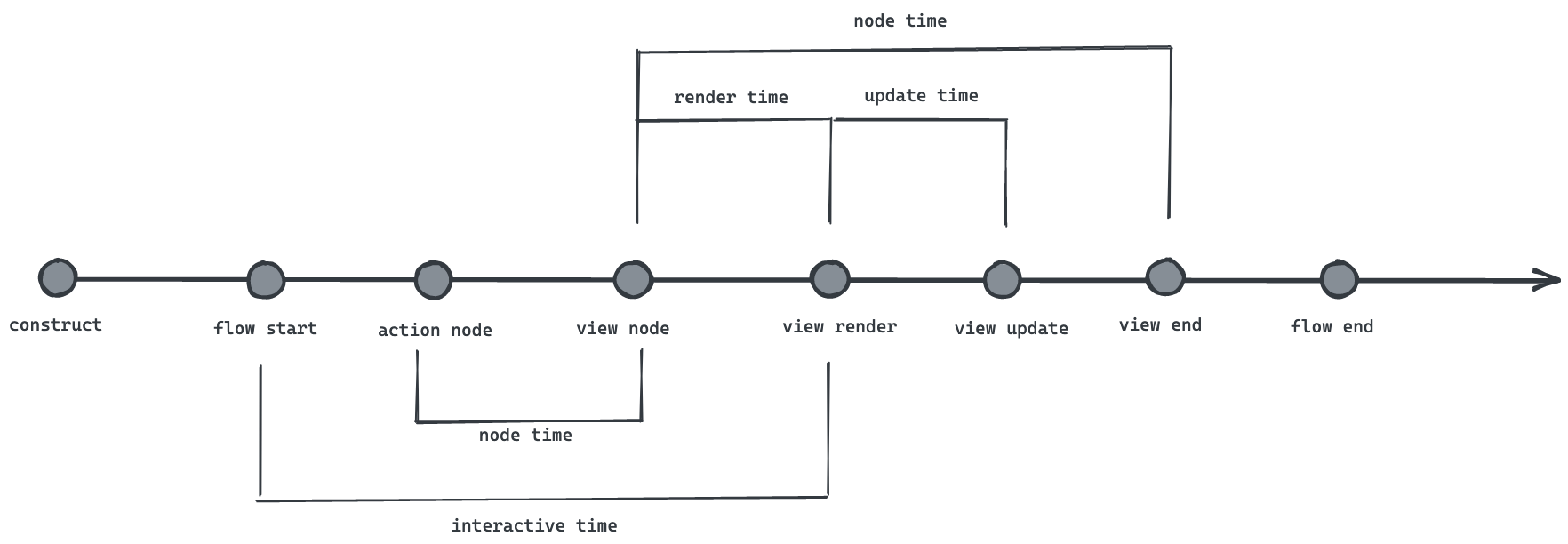
Usage
Add the plugin to Player:
import { Player } from '@player-ui/player'; import { MetricsPlugin } from '@player-ui/metrics-plugin'; const player = new Player({ plugins: [ new MetricsPlugin({ onUpdate: (metrics) => { // Handle the update } }) ] })
The
onUpdate
callback will be invoked for any update to the metrics. There are also callbacks for finer-grained events (onRenderEnd
, onInteractive
, etc), as well as a hooks
based API for even more control.Using a custom timer
By default, all time is measured in ms using
performance.now()
with a fallback to the less-accurate Date.now()
.
If you wish to supply your own timer, simply use the getTime
option to set the function to use.Measuring Render Time
For extensions of this plugin that wish to track the render (and update) times of nodes, add the
trackRenderTime
flag to options
. You must then call metrics.renderEnd()
to denote when content is painted on the screen. This is automatically handled for the platform specific versions of this plugin.The
react
version of the Metrics Plugin adds support for render
and update
times to events. The API mirrors that of the core version:import { ReactPlayer } from '@player-ui/react'; import { MetricsPlugin } from '@player-ui/metrics-plugin-react'; const player = new ReactPlayer({ plugins: [ new MetricsPlugin({ onUpdate: (metrics) => { // Handle the update } }) ] })
The
ios
version of the Metrics Plugin will track initial render time for each view in a flow. Due to current SwiftUI limitations, update time can’t be tracked yet. It can be used in conjunction with a core plugin that utilizes the events, through findPlugin
, or standalone.CocoaPods
Add the subspec to your
Podfile
pod 'PlayerUI/MetricsPlugin'
Swift Usage
var body: some View { SwiftUIPlayer( flow: flow, plugins: [ // Tracking render time can be controlled with a parameter MetricsPlugin(trackRenderTime: true) { timing, nodeMetrics, flowMetrics in // Handle metrics payload log(timing.duration ?? -1) } ], result: $resultBinding ) }
The
JVM
Metrics plugin can track render time for views in a flow.In build.gradle
implementation "com.intuit.player.plugins:metrics:$PLAYER_VERSION"
In Player constructor
import com.intuit.player.plugins.expression.ExpressionPlugin val metricsPlugin = MetricsPlugin { timing, renderMetrics, flowMetrics -> ... } AndroidPlayer(metricsPlugin)
Beaconing
The Metrics Plugin also includes a plugin for the Beacon Plugin that adds render time to the hook context for
viewed
beacons send for views. This plugin is automatically registered to the Beacon Plugin if the trackRenderTime
option is enabled.In order to actually include the render-time in a beacon, you must create a
BeaconPluginPlugin
that maps the renderTime from the hook’s context to the actual beacon object. It can be accessed through the MetricsViewBeaconPluginContextSymbol
key:import { MetricsViewBeaconPluginContextSymbol } from '@player-ui/metrics-plugin'; import { BeaconPluginPlugin } from '@player-ui/beacon-plugin'; class MyBeaconPluginPlugin implements BeaconPluginPlugin { apply(beaconPlugin: BeaconPlugin) { beaconPlugin.hooks.buildBeacon.tap( { name: 'my-beacon-plugin', context: true } as Tap, async (context, beacon) => { const { renderTime } = (await (context as any)[MetricsViewBeaconPluginContextSymbol]) || {}; return { ...beacon, ...(renderTime && { renderTime }), }; } ); }
See the Beacon Plugin for more info.